Minesweeper Solver
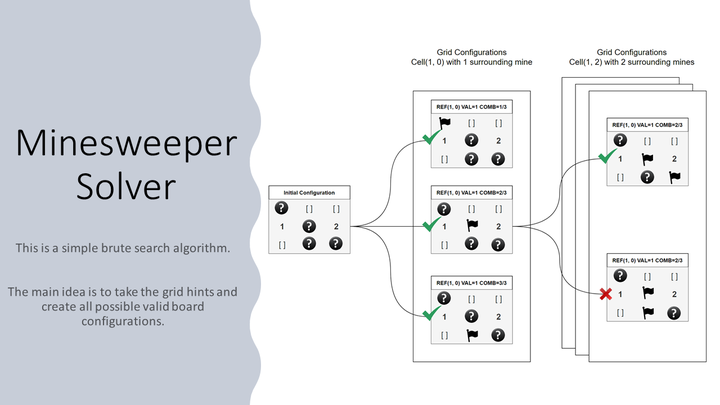
This simple python implementation is able to infer the solution from a minesweeper board. Consider the following board configuration:
We can respresent that board on the commandline by using a [.]
notation. You should be able to get a solution by running:
$ minesweeper --timeout 3 --grid """
[2] [?] [1] [?]
[?] [?] [3] [?]
[3] [?] [?] [?]
[?] [1] [?] [1]
"""
Expected output:
[2] [ ] [1] [ ]
[*] [*] [3] [ ]
[3] [*] [ ] [*]
[ ] [1] [ ] [1]
Installation
Install this application using pip directly referencing the dev branch from github:
$ pip install git+https://github.com/rhdzmota/minesweeper.git@dev#subdirectory=minesweeper&egg=minesweeper
Alteratively, you can clone this repository and install the minesweeper package with:
$ pip install -e minesweeper
Verify correct installation by running: minesweeper --help
or python -m minesweeper --help
CLI Usage
Provide a grid with the following cell types:
[ ]
: empty cell.[?]
: non-visible cell.[*]
: flagged cell.[i]
: cell with a number hint between 1 to 8 (e.g., [3], [1]).
You can solve any board configuration by running the --grid
command:
$ minesweeper --timeout 3 --grid """
[3] [?] [2] [?]
[?] [?] [ ] [?]
"""
Expected output:
```text
[3] [*] [2] [ ]
[*] [*] [ ] [ ]
Package Usage
Once installed, you can also use this application as a python library.
Create a simple board:
from minesweeper.board import Grid, Cell
my_grid = Grid(
values=[
[Cell.num(2), Cell.unk(), Cell.num(1), Cell.unk()],
[Cell.unk(), Cell.unk(), Cell.num(3), Cell.unk()],
[Cell.num(3), Cell.unk(), Cell.unk(), Cell.unk()],
[Cell.unk(), Cell.num(1), Cell.unk(), Cell.num(1)]
]
)
my_grid.show()
You can solve a grid by using the Solver
class:
from minesweeper.solver import Solver
solver = Solver(gird=my_grid)
solver.run(timeout=10)
if solver.finished():
solution = solver.final_state
solution.show()